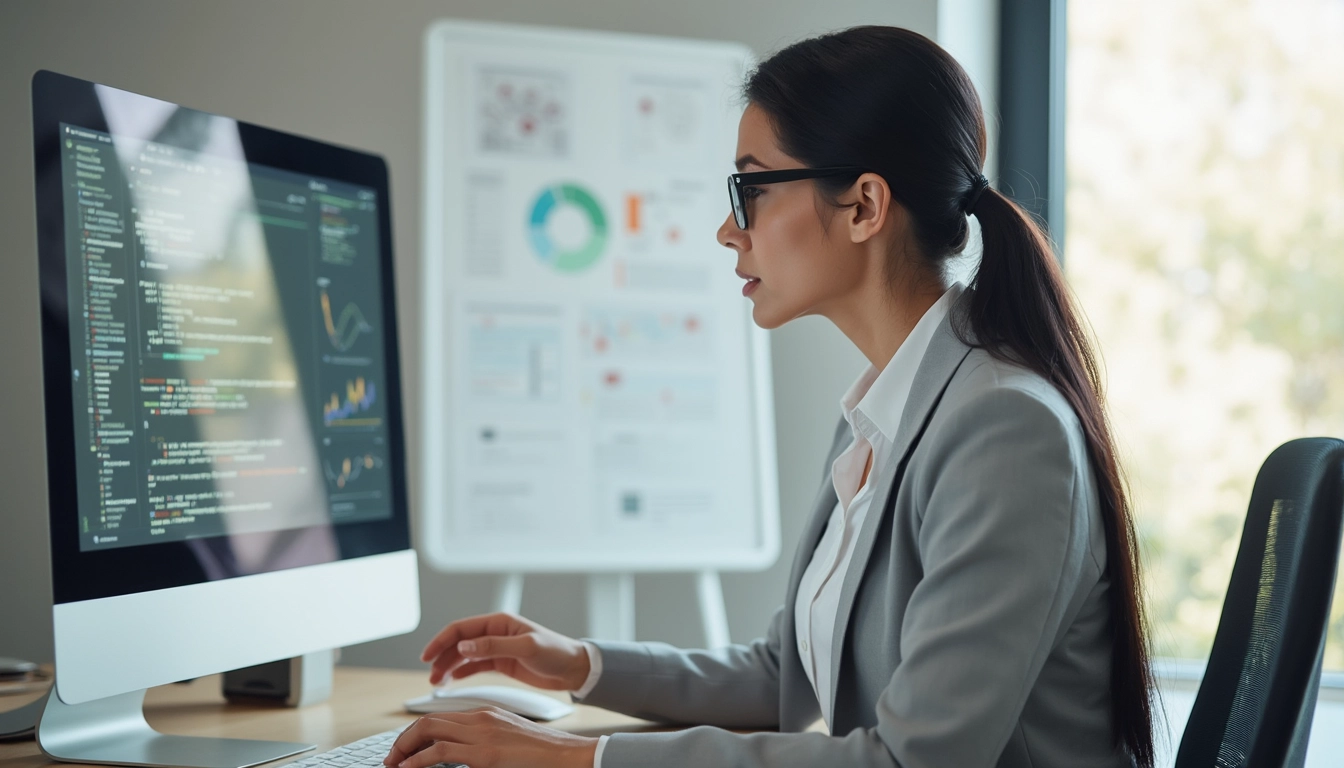
Mastering JSON: Essential Guide for Web Developers and API Designers
JSON (JavaScript Object Notation) has become the lingua franca of data exchange on the internet. This lightweight, text-based format offers a simple yet powerful way to represent structured data, making it essential for web developers and API designers alike.
Key takeaways:
- JSON is a human-readable data format used for storing and transmitting information
- It uses key-value pairs and supports various data types including strings, numbers, and arrays
- JSON is language-independent, making it versatile for different programming environments
- It’s widely used in web development and API communication
- Understanding JSON is crucial for working with modern web applications and data interchange
What is JSON and Why is it Important?
JSON, short for JavaScript Object Notation, is a lightweight data interchange format that has taken the web development world by storm. Originally developed for JavaScript, JSON has transcended its roots to become a universal language for data exchange across various programming environments.
The importance of JSON lies in its simplicity and readability. It uses a structure of key-value pairs that’s easy for humans to read and write, while also being simple for machines to parse and generate. This dual nature makes JSON an ideal format for data transmission between a server and a web application.
Structure and Syntax of JSON
At its core, JSON is built on two structures:
- A collection of name/value pairs (realized as an object in most languages)
- An ordered list of values (realized as an array in most languages)
The basic syntax of JSON involves enclosing data in curly braces {} for objects and square brackets [] for arrays. Here’s a simple example of a JSON object:
{“name”: “Katherine Johnson”, “age”: 101, “city”: “Newport News”}
This structure is intuitive and maps easily to data structures in most programming languages, making JSON a versatile choice for data interchange.
JSON Data Types in Detail
JSON supports several data types, allowing for rich and complex data structures:
- Strings: Text enclosed in double quotes, e.g., “Hello, World!”
- Numbers: Integer or floating-point, e.g., 42 or 3.14
- Booleans: true or false
- Arrays: Ordered lists of values, e.g., [1, 2, 3] or [“Red”, “Green”, “Blue”]
- Objects: Unordered collections of key-value pairs
- Null: Representing an empty or non-existent value
This variety of data types allows JSON to represent most common data structures used in programming, making it a flexible format for diverse applications.
Working with JSON in Programming
In the world of web development, JSON plays a crucial role in API communication. When you make a request to a web API, the response is often in JSON format. To work with this data in JavaScript, you’ll commonly use two methods:
- JSON.parse(): Converts a JSON string into a JavaScript object
- JSON.stringify(): Converts a JavaScript object into a JSON string
For example, to parse a JSON string:
const person = JSON.parse(‘{“name”: “John”, “age”: 30}’);
This versatility in handling data makes JSON an essential tool for automating data processing tasks in web applications.
Best Practices and Tools for JSON
When working with JSON, it’s important to follow some best practices:
- Validate your JSON: Use tools like JSONLint to ensure your JSON is valid
- Use consistent naming conventions: Stick to camelCase or snake_case for key names
- Format for readability: Use line breaks and indentation for complex structures
- Be mindful of data types: Ensure you’re using the correct data types for your values
These practices will help you create clean, maintainable JSON that’s easy to work with and less prone to errors.
Practical Applications and Learning Resources
JSON’s simplicity and flexibility make it ideal for various applications beyond just data transfer. It’s commonly used for:
- Configuration files in applications
- Storing application settings
- Serializing and deserializing data structures
- Mock data for testing and development
To deepen your understanding of JSON, consider exploring comprehensive guides and tutorials available online. Automation platforms often provide excellent resources for working with JSON in practical scenarios, helping you apply your knowledge to real-world problems.
By mastering JSON, you’ll equip yourself with a valuable skill that’s essential in modern web development and data processing. Whether you’re building web applications, working with APIs, or managing configuration files, a solid grasp of JSON will serve you well in your programming journey.
Sources:
Stack Overflow Blog – “A Beginner’s Guide to JSON: The Data Format for the Internet”
Nylas Blog – “Complete Guide to Working with JSON”
Bedrock Wiki – “Understanding JSON”
RGB Studios Blog – “A Beginner’s Guide to JSON”
DigitalOcean Community – “An Introduction to JSON”