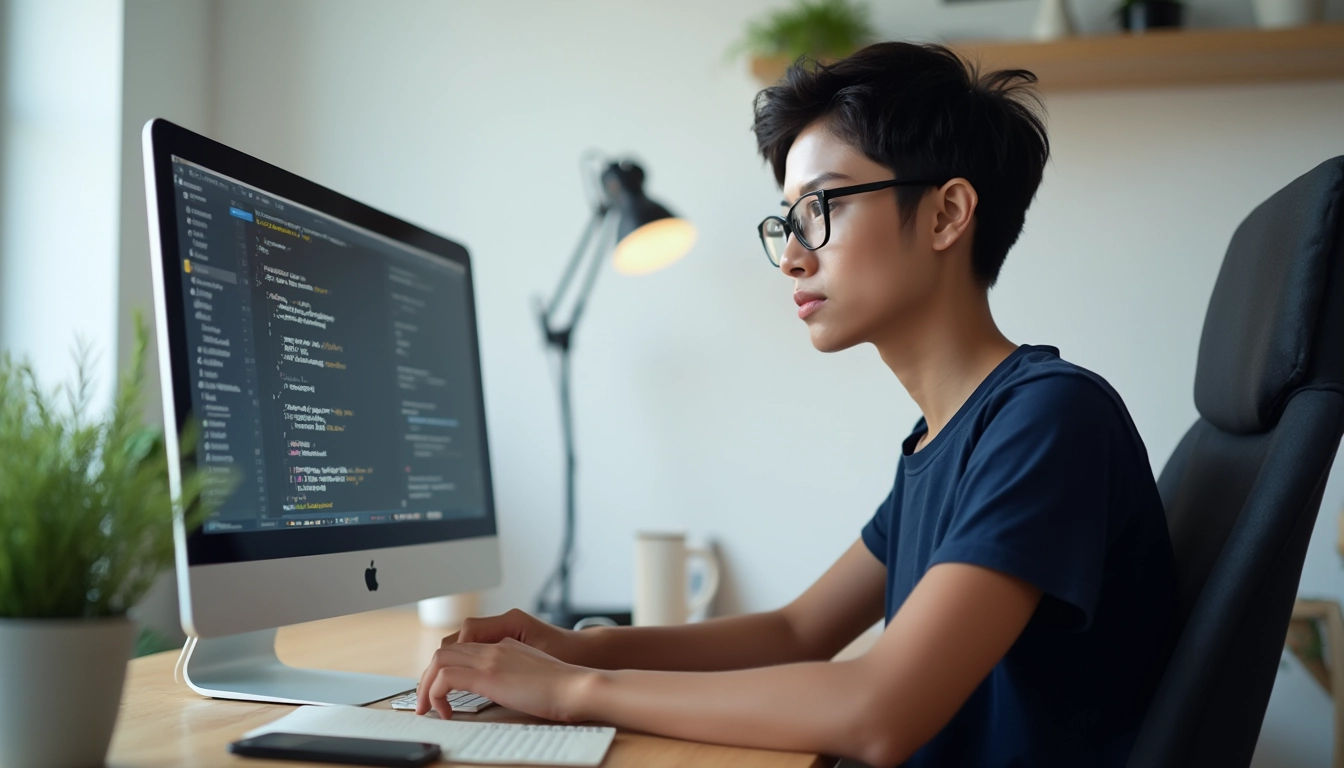
Mastering JSON: A Comprehensive Guide to Syntax and Implementation
JSON (JavaScript Object Notation) is a lightweight data interchange format that has become a cornerstone of modern web development. Its simplicity and versatility make it an essential tool for storing and transmitting structured data between servers and web applications.
Key takeaways:
- JSON is a text-based, human-readable format derived from JavaScript object notation syntax
- It uses key-value pairs and arrays to represent data structures
- JSON is language-independent and compatible with many programming environments
- Its lightweight nature makes it ideal for data transfer in web applications
- JSON has a simple syntax that’s easy to read, write, and parse
Table of Contents
Understanding JSON Syntax and Structure
At its core, JSON uses a straightforward structure based on two main concepts: objects and arrays. Objects are enclosed in curly brackets {} and contain key-value pairs, while arrays are enclosed in square brackets [] and contain ordered lists of values.
Here’s a basic example of JSON syntax:
{
“name”: “John Doe”,
“age”: 30,
“city”: “New York”
}
In this example, we have an object with three key-value pairs. The keys are always strings, while the values can be of various data types.
JSON Data Types
JSON supports several data types, making it flexible enough to represent a wide range of information:
- Strings: Text enclosed in double quotes
- Numbers: Integer or floating-point
- Booleans: true or false
- Null: Represents a null value
- Objects: Collections of key-value pairs
- Arrays: Ordered lists of values
Practical JSON Examples
Let’s look at a more complex JSON example to illustrate its capabilities:
{
“className”: “Class 2B”,
“year”: 2022,
“active”: true,
“homeroomTeacher”: {
“firstName”: “Richard”,
“lastName”: “Roe”
},
“members”: [
{“firstName”: “Jane”, “lastName”: “Doe”},
{“firstName”: “Jinny”, “lastName”: “Doe”},
{“firstName”: “Johnny”, “lastName”: “Doe”}
]
}
This example showcases nested objects and arrays, demonstrating how JSON can represent complex data structures. It’s worth noting that JSON’s flexibility allows for easy integration with various programming languages and frameworks.
Advantages of Using JSON
JSON has gained widespread adoption due to several key advantages:
- Human-readable: Its simple structure makes it easy to understand and debug
- Lightweight: JSON is more compact than XML, reducing data transfer overhead
- Language-independent: It can be used with most modern programming languages
- Fast parsing: Many languages have built-in support for parsing JSON quickly
These benefits make JSON an excellent choice for data interchange in web applications and APIs. Its simplicity and efficiency have contributed to its widespread use in modern web development.
JSON Cheat Sheet: Quick Reference Guide
To help you get started with JSON, here’s a quick reference guide:
- Object syntax: {“key”: value, “key”: value}
- Array syntax: [“value1”, “value2”, “value3”]
- String: “text”
- Number: 42 or 3.14
- Boolean: true or false
- Null: null
Remember that JSON requires double quotes for strings and doesn’t support comments. When working with JSON in your projects, always ensure proper formatting and validate your JSON to avoid errors.
Conclusion
JSON has revolutionized data interchange in web development. Its simplicity, readability, and wide support across programming languages make it an indispensable tool for modern developers. Whether you’re building APIs, working with databases, or handling configuration files, understanding JSON is crucial for efficient and effective development.
As you continue to work with JSON, you’ll find that its flexibility allows for creative solutions to various data representation challenges. Don’t hesitate to explore more advanced JSON concepts and best practices to make the most of this powerful data format in your projects.
Sources:
– Hostinger
– W3Schools
– TheServerSide
12 thoughts on “Mastering JSON: A Comprehensive Guide to Syntax and Implementation”