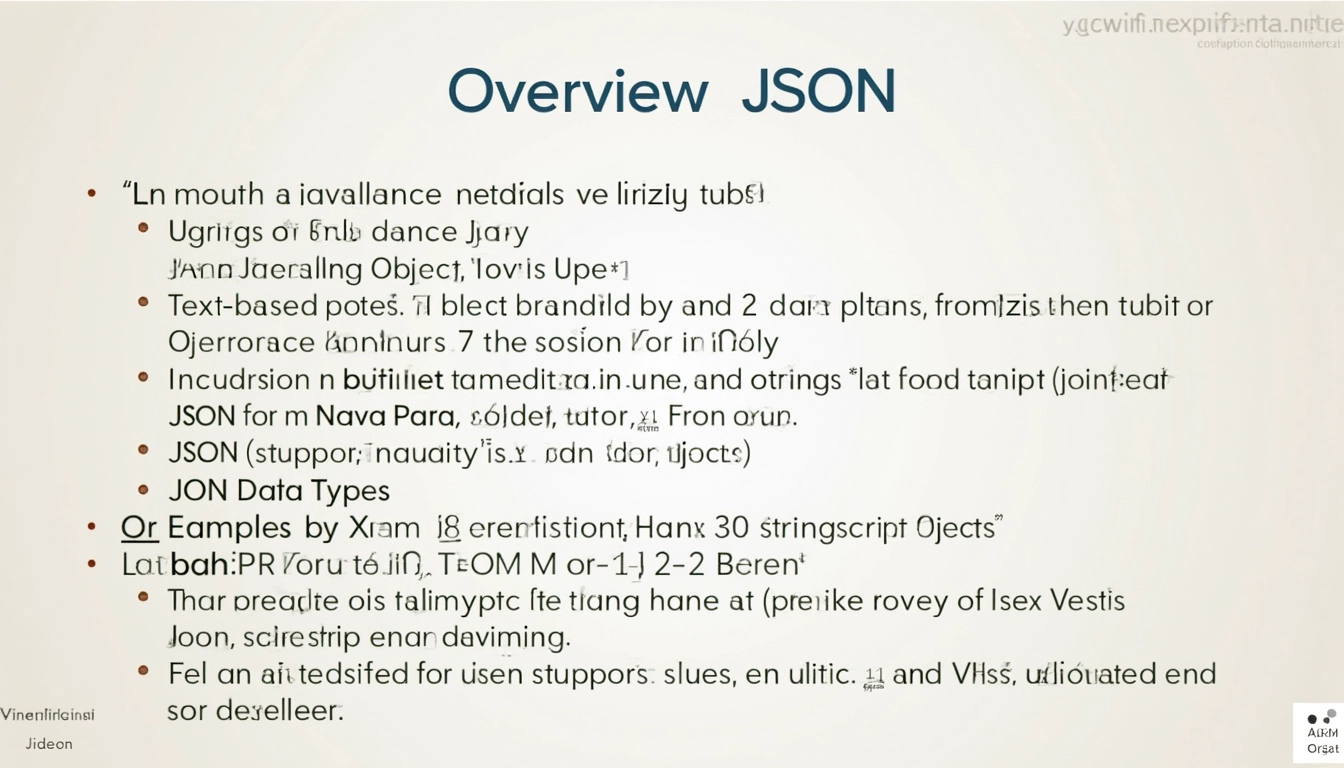
Mastering JSON: A Comprehensive Guide to Syntax, Usage, and Best Practices
JSON, or JavaScript Object Notation, is a lightweight and versatile data interchange format that has become a cornerstone of modern web development. Its simplicity and flexibility make it an indispensable tool for storing and transmitting structured data across various platforms and programming languages.
Key Takeaways:
- JSON is a text-based data format derived from JavaScript object syntax
- It uses key-value pairs and arrays to represent data structures
- JSON supports various data types including strings, numbers, booleans, and null
- Its lightweight nature makes it ideal for data interchange in web applications
- JSON is language-independent and widely supported across platforms
Understanding JSON Syntax
JSON’s syntax is straightforward and easy to read. It consists of two primary structures: objects and arrays. Objects are enclosed in curly braces {} and contain key-value pairs, while arrays are enclosed in square brackets [] and contain an ordered list of values.
Here’s a simple example of a JSON object:
{ "name": "John Doe", "age": 30, "city": "New York" }
In this example, we have an object with three key-value pairs. The keys are always strings enclosed in double quotes, followed by a colon, and then the corresponding value. Automating the creation and parsing of JSON data can significantly streamline your workflow, especially when dealing with large datasets.
JSON Data Types
JSON supports several data types, making it flexible for various use cases. The supported data types include:
- Strings: Text enclosed in double quotes
- Numbers: Integer or floating-point
- Booleans: true or false
- null: Represents a null value
- Objects: Collections of key-value pairs
- Arrays: Ordered lists of values
This variety of data types allows JSON to represent complex data structures efficiently. For instance, you can have arrays of objects, nested objects, or even arrays within objects.
Working with JSON in JavaScript
One of the great advantages of JSON is its seamless integration with JavaScript. You can easily convert JSON strings to JavaScript objects and vice versa using built-in methods:
- JSON.parse(): Converts a JSON string to a JavaScript object
- JSON.stringify(): Converts a JavaScript object to a JSON string
These methods make it simple to work with data received from APIs or stored in JSON format. Once parsed, you can access and manipulate the data using dot notation or bracket notation, just like any other JavaScript object.
JSON in Data Interchange
JSON’s popularity in data interchange stems from its lightweight nature and ease of use. It’s commonly used in RESTful APIs, configuration files, and as a data storage format. The simplicity of JSON makes it an excellent choice for transmitting data between a server and a web application.
When working with APIs, JSON is often the preferred format for sending and receiving data. Its structure allows for easy serialization and deserialization, making it efficient for both client-side and server-side processing.
JSON vs. Other Data Formats
While JSON is widely used, it’s not the only data interchange format available. XML (eXtensible Markup Language) is another popular option. However, JSON has several advantages over XML:
- JSON is more concise and easier to read
- It has a simpler structure, making it faster to parse
- JSON is natively supported in JavaScript, reducing the need for additional parsing libraries
These factors contribute to JSON’s widespread adoption in modern web development and data exchange scenarios.
Best Practices for Using JSON
To make the most of JSON in your projects, consider the following best practices:
- Use clear and descriptive key names for better readability
- Keep your JSON structures as flat as possible to improve performance
- Validate your JSON to ensure it’s well-formed before using it
- Use appropriate data types for your values (e.g., numbers for numeric data instead of strings)
By following these guidelines, you’ll create more efficient and maintainable JSON structures in your applications.
Conclusion
JSON has become an essential part of web development due to its simplicity, flexibility, and wide support across platforms. Understanding JSON syntax and best practices is crucial for any developer working with web technologies or data interchange. As you continue to work with JSON, you’ll find it an invaluable tool in your development toolkit, enabling efficient data storage, transmission, and manipulation in various applications and systems.
Sources:
Hostinger
W3Schools
JSON-5
Code Institute