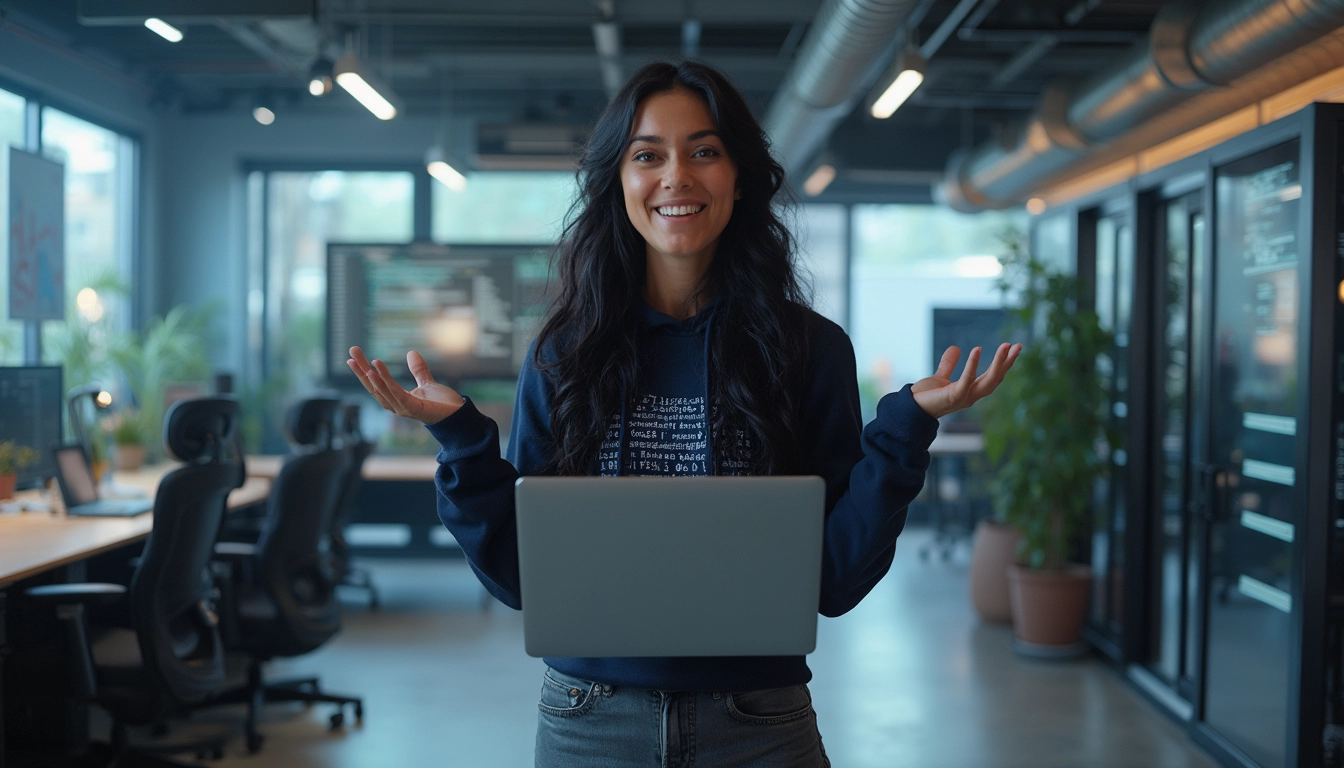
Mastering JSON: A Comprehensive Guide for Modern Web Developers
JSON, or JavaScript Object Notation, is a lightweight data interchange format that has become ubiquitous in modern web development. It provides a simple yet powerful way to represent structured data, making it an essential tool for developers working with APIs and data storage.
Key Takeaways:
- JSON uses a human-readable format consisting of key-value pairs and arrays
- It supports six data types: strings, numbers, booleans, arrays, objects, and null
- JSON can be easily parsed and stringified in JavaScript using built-in functions
- It’s widely used in APIs, web applications, and data storage scenarios
- Tools like JSONLint and JSON Server can help with validation and API creation
Understanding JSON Structure
At its core, JSON is built around two main structures: objects and arrays. Objects are enclosed in curly braces {} and contain key-value pairs, while arrays are enclosed in square brackets [] and contain ordered lists of values. Let’s look at a simple JSON object:
{
“name”: “John Doe”,
“age”: 30,
“city”: “New York”,
“isStudent”: false,
“hobbies”: [“reading”, “cycling”, “photography”]
}
This example demonstrates the various data types supported by JSON: strings, numbers, booleans, and arrays. JSON’s simplicity and flexibility make it an ideal choice for data exchange between different systems and programming languages.
Working with JSON in JavaScript
JavaScript provides built-in functions to work with JSON data. The JSON.parse() method converts a JSON string into a JavaScript object, while JSON.stringify() does the opposite, converting a JavaScript object into a JSON string. Here’s how you can use these functions:
// Converting JSON string to JavaScript object
const jsonString = ‘{“name”: “Alice”, “age”: 25}’;
const person = JSON.parse(jsonString);
console.log(person.name); // Output: Alice
// Converting JavaScript object to JSON string
const car = {make: “Toyota”, model: “Camry”, year: 2022};
const carJson = JSON.stringify(car);
console.log(carJson); // Output: {“make”:”Toyota”,”model”:”Camry”,”year”:2022}
These functions are crucial when working with APIs or storing data in JSON format in JavaScript. They allow you to easily manipulate and transform data between JSON and JavaScript objects.
JSON in APIs and Data Exchange
JSON has become the de facto standard for data exchange in modern web applications and APIs. Its lightweight nature and ease of use make it perfect for transmitting data over networks. When working with APIs, you’ll often send and receive data in JSON format. Here’s an example of how you might fetch data from an API using JavaScript’s fetch function:
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(‘Error:’, error));
In this example, the response.json() method parses the JSON response from the API into a JavaScript object, making it easy to work with the received data.
Validating and Formatting JSON
When working with JSON, it’s crucial to ensure that your data is valid and well-formatted. Invalid JSON can cause errors and break your application. Tools like JSONLint can help you validate your JSON and identify any syntax errors. For formatting, you can use online tools or integrated development environment (IDE) extensions to make your JSON more readable.
Here’s an example of poorly formatted JSON:
{“name”:”John”,”age”:30,”city”:”New York”}
And here’s the same JSON, properly formatted:
{
“name”: “John”,
“age”: 30,
“city”: “New York”
}
Proper formatting improves readability and makes it easier to spot errors or make changes to your JSON data.
Creating Mock APIs with JSON Server
For development and testing purposes, you might need to create a mock API quickly. This is where JSON Server comes in handy. It’s a Node.js module that allows you to create a full fake REST API with zero coding in less than 30 seconds. Here’s how you can set it up:
1. Install JSON Server globally:
npm install -g json-server
2. Create a db.json file with some data:
{
“posts”: [
{ “id”: 1, “title”: “json-server”, “author”: “typicode” }
],
“comments”: [
{ “id”: 1, “body”: “some comment”, “postId”: 1 }
]
}
3. Start JSON Server:
json-server –watch db.json
Now you have a full REST API that you can use for development and testing. This can be incredibly useful when you’re building a front-end application and need to simulate API responses.
Conclusion
JSON has revolutionized the way we handle data in web development. Its simplicity, flexibility, and widespread support make it an indispensable tool for modern developers. Whether you’re working with APIs, storing data, or creating mock services, understanding JSON is crucial for efficient and effective development.
If you’re looking to automate your JSON-related tasks or any other aspect of your development workflow, I recommend checking out Make.com. It’s a powerful automation platform that can help streamline your processes, including working with APIs and JSON data.
By mastering JSON and leveraging tools like JSON Server and automation platforms, you’ll be well-equipped to handle data effectively in your web development projects.
Sources:
An Introduction to JSON
How to Use JSON.Parse and JSON.Stringify
How to Transform JSON Data with JQ
How to Work with JSON in JavaScript
JSON Server